Module 18 - Introduction to Loops
What are Loops
Loops allow for repetitive or iterative tasks, and can save a lot of time and code. Each iteration can vary in their variables, values, and conditions. There are different types of loops in JavaScript, and they have small differences, but essentially do the same thing: loop over data.
Loops are used to run through a set of instructions in the code a specific number of times. With a loop, you can tell the code to perform the same task, or series of tasks, multiple times.
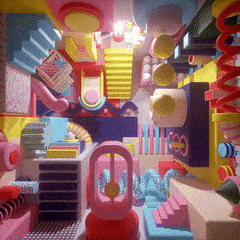
For Loop
Before moving forward, take a moment to dig deeper into the anatomy of the for loop. Check out the example below. What's this all about?
for (initialExpression; condition; incrementExpression) {
loop body
}
The for loop requires those 3 parts to iterate: - counter A variable that is typically initialized with a number that counts the number of iterations. - condition Expression that uses comparison operators to cause the loop to stop when true - increment-expression Runs at the end of each iteration, typically used to change the counter value
//Counting up to 10
for (let i = 0; i < 10; i++) {
console.log(i);
}
✅ Run this code in repl.it. What happens when you make small changes to the counter, condition, or iteration expression?
This code below script just prints the number of times that the loop has run.
// another example of a for loop
let countMe = 4;
for (let step = 1; step <= countMe; step++) {
console.log(step);
}
This code will log the numbers 1 through 5 to the console.
- Starting at step = 1, you can follow along:
- Check if step is less than or equal to the value of countTo (4).
- Log the value of step.
- Increase step by one.
- Then repeat this code.
You'll also notice the curly brackets {} used here. The } at the end of the loop tells the code to run the loop and to keep running it—until the condition is false.
I, We, You Do
In the Repl.it below, practice:
- Write a for loop that logs the numbers from 1 to 5.
- Write a for loop that logs the numbers from 5 to 10. (Hint: Change the initial value of the counter.)
- Write a for loop that logs the numbers from 0 to 50,
- counting up by 10. (Hint: Change the increment.) Hint: The loop body should be the same. You should only need to change what's in the parentheses!
// Write a for loop that logs the numbers up to 5
let countMe = 5;
for (let i = 1; i <= countTo; i++) {
console.log(i);
}
// Write a for loop that logs the numbers from 5 to 10
let newCountMe = 10;
// Write a for loop that logs the numbers from 0 to 50, counting by 10s
let longCountMe = 50;
Loops and Arrays
Arrays are often used with loops because most conditions require the length of the array to stop the loop, and the index can also be the counter value.
let iceCreamFlavors = ["Chocolate", "Strawberry", "Vanilla", "Pistachio", "Rocky Road"];
for (let i = 0; i < iceCreamFlavors.length; i++) {
console.log(iceCreamFlavors[i]);
} //Ends when all flavors are printed
✅In repl, copy and paste and then experiment with looping over an array on your own
Take Home Practice
1. Write a for loop that adds up all the numbers in the array to the totalSnacksEaten variable.
// don't modify these variables
let snacksEatenPerDay = [3, 2, 5, 6, 1, 2, 2, 4, 8, 2, 5, 3, 3, 1];
let totalSnacksEaten = 0;
// write a for loop that adds up all the numbers in the array to the totalSnacksEaten variable
Module 19- Intro to Objects

An object is a type of collection that holds information, just like an array. But instead of operating like a storage box, an object can be represented using another analogy. Objects are like a collection of gifts that need to be handed out to a group of people.
An object holds many values (represented by the gifts), and each value has a unique key (represented by the gift tags). Let's model the gift example below using an object.
// an example of an object
let gifts = {
tommy: "stuffed giraffe",
lisa: "book",
roberto: "cap",
beth: "suit of armor",
};
What you see here is a JavaScript object. It has the unique gift tags, as you see above—which are known as keys or properties in JavaScript. And it has the gifts themselves—the book and cap- are called values. Objects allow you to keep track of the value that belongs to each key. Then, you can retrieve a value using the name of the key.
Object syntax
As seen above, Objects start and end with curly brackets: the { and }. Keys must be a string data type, but they can be any string. A colon : sits between each key and the value it points to, and a comma , separates key: value pairs.
Unlike keys, values can be any data type. They can be a number, an array, a boolean value, null, or even another object. Any JavaScript data type can be a value. Take a look at the example below.
// an Object with a mix of data types
let actionFigure = {
name: "Super Awesome Guy",
heightInCentimeters: 10,
accessories: ["cape", "disguise", "laser ring"],
limitedEdition: true,
};
Dot notation
Once an object is defined, you can get the values inside it using either dot notation or bracket notation. Dot notation is the most common method for retrieving values from inside an object, and it looks like this: objectName.key. As you can see, this notation uses a period ., or dot. In the example below, can you see the dot notation?
let actionFigure = {
name: "Super Awesome Guy",
heightInCentimeters: 10,
accessories: ["cape", "disguise", "laser ring"],
limitedEdition: true,
};
actionFigure.name; // 'Super Awesome Guy'
actionFigure.limitedEdition; // true
I, You, We do exercise
Accessing values inside an object It's time for a demo. Play around with this REPL to practice accessing values inside an object.
let myFavorites = {
movie: "Shrek",
song: "The Rhapsody",
food: "Spaghetti",
drink: "Sprite"
};
console.log(myFavorites.movie); // 'Shrek'
Imagine you don't want your friends to know that your grades are suffering, so go ahead and "hack" your grades! In the REPL below, change the test with the lowest score to 88.
let myGrades = {
test0: 100,
test1: 68,
test2: 98.5,
test3: 90,
};
// Modify the object using dot notation below
console.log(myGrades);
Bracket notation
You'll sometimes need to use bracket notation when the key you wish to access is a string with a space in it. In this case, the key won't work as a dot accessor. You have to use a bracket notation.
Examples
Here are some exercies to help you practice this.
// getting information out of an object
let categories = {
'best picture': 'Shrek',
'best director': 'Shrek 2'
};
// we can't do these
categories.best director;
categories.best picture;
// so we use bracket notation
categories['best picture']; // 'Shrek'
categories['best director']; // 'Shrek 2'
Adding key–value pairs to an object
Once you've defined an object, you can add new key–value pairs to the object using either dot notation or bracket notation. Here's an example of how to add a key and an associated value to an object. Which key–value pairs are being added to this object?
// adding a new key/value pair
let myFamily = {
mom: "Cynthia",
dad: "Paul",
};
myFamily.sister = "Lucinda"; // dot notation
myFamily["brother in law"] = "Merle"; // bracket notation
Then update an existing key's value with the same syntax, like in the example below.
myFamily["brother in law"] = "Scotty";
What is Accumulator Pattern
The accumulator pattern is a particularly important pattern for you to have in your developer's toolkit. The accumulator pattern is a method of building up or accumulating data. And as you'll see, it's very useful.

Accumulator sum
Play around with this and see what happens.
let countTo = 10; // how high should we count?
let sum = 0; // this is a place to store our sum
for (let i = 1; i <= countTo; i+=2) {
sum += i;
console.log("The current sum is: " + sum);
}
console.log("The final sum is: " + sum);
In the example above, the code combined many numbers into a single number. How would you apply this idea to other situations? How would you combine several strings into a single string?
// an example of the accumulator pattern
let colors = ["red", "white", "blue"];
let statement = "My favorite colors are ";
for (let i = 0; i < colors.length; i++) {
// what could you write here?
}
// challenge 1 - make the statement say:
// 'My favorite colors are red, white, blue,'
// challenge 2 - make the statement say:
// 'My favorite colors are red, white and blue.'
console.log(statement);
Specifically, the for loop is checking whether each object has attending set to true or false<. This loop uses almost every concept you have seen so far: variables, control flow, loops, arrays, and objects. Read the code carefully before running it, and try to predict what it will do.
Take Home Practice
let partyPeople = [
{ name: "Joe", attending: false },
{ name: "Jasmine", attending: true },
{ name: "Julio", attending: false },
{ name: "Julia", attending: true },
];
// loop through each item
// check if the person is attending
// if they are attending, say they are partying
// otherwise, say they are burnt out
Module 20 - Writing Functions
At its core, a function is a block of code we can execute on demand. This is perfect for scenarios where we need to perform the same task multiple times; rather than duplicating the logic in multiple locations (which would make it hard to update when the time comes), we can centralize it in one location, and call it whenever we need the operation performed - you can even call functions from other functions!

Creating and calling a function
function nameOfFunction() { // function definition
// function definition/body
}
If I wanted to create a function to display a greeting, it might look like this:
function displayGreeting() {
console.log('Hello, world!');
}
When we want to call our function and pass in the parameter, we specify it in the parenthesis.
displayGreeting('Christopher');
// displays "Hello, Christopher!" when run
Default values
We can make our function even more flexible by adding more parameters. But what if we don't want to require every value be specified? Keeping with our greeting example, we could leave name as required (we need to know who we're greeting), but we want to allow the greeting itself to be customized as desired. If someone doesn't want to customize it, we provide a default value instead.
To provide a default value to a parameter, we set it much in the same way we set a value for a variable -
parameterName = 'defaultValue'.To see a full example:
function displayGreeting(name, salutation='Hello') {
console.log(`${salutation}, ${name}`);
}
When we call the function, we can then decide if we want to set a value for salutation.
displayGreeting('Christopher');
// displays "Hello, Christopher"
displayGreeting('Christopher', 'Hi');
// displays "Hi, Christopher"
Return values
A return value is returned by the function, and can be stored in a variable just the same as we could store a literal value such as a string or number. If a function does return something then the keyword return is used. The return keyword expects a value or reference of what's being returned like so:
return myVariable;
We could create a function to create a greeting message and return the value back to the caller
function createGreetingMessage(name) {
const message = `Hello, ${name}`;
return message;
}
Arguments and Parameters
Some functions need you to give them something to work properly. When we define a function we can include a parameter.
function favoriteFood(food) {
console.log("My favorite food is " + food);
}
//favoriteFood("plantain");
The function above needs a string to work properly so we created a placeholder called food. In programming, we call these placeholders parameters. In the example above we invoked the favoriteFood function and passed it the string "plantain"- which is what is known as an argument. When we pass arguments to our function, they are matched with the appropriate parameter and substituted in. For the favoriteFood function, food will be "plantain" this time, since that's what we gave to the function.
Say hello to my friend
Write a function called sayHelloToMyFriend that logs
"Hello, {the name of the friend}, it's nice to meet you!".
It should take in one argument (a string with the friend's name). It should use the + operator to add strings together, and use console.log to print the full sentence. For example 1:
sayHelloToMyFriend("Deniz") //-> "Hello, Deniz, it's nice to meet you!"
sayHelloToMyFriend("Eddie") //-> "Hello, Eddie, it's nice to meet you!"
sayHelloToMyFriend("Alaya") //-> "Hello, Alaya, it's nice to meet you!"
function sayHelloToMyFriend(Hello) {
console.log(Hello + "," + " it's nice to meet you!");
}
sayHelloToMyFriend("Hello, Deniz");
sayHelloToMyFriend("Hello, Eddie");
sayHelloToMyFriend("Hello, Alaya");
<>
Passing An Argument
When you define a function it can have a parameter. Let's look at the example A - function sayHello.
function sayHello(name) {
console.log("Hello, " + name + "!");
}
sayHello("James");
So we defined a function that takes a string and we invoked it with the argument "James." Example B:
function sayHello(name) {
console.log("Hello, " + name + "!");
}
let myFriend = "George";
sayHello(myFriend);
In both examples, the function hasn't changed at all. What's different is that we created a variable called myFriend and we passed that in as an argument. The contents of myFriend is the string "James." It's the exact same thing as earlier, we are just using the variable as an extra step.
Functions and Loops
Functions can do more than just log values, calculate numbers, and change strings.
Loops review using accumulator pattern
We can use for loops to iterate through a collection of items. If we had a collection of numbers we could add them all up.
let goalsScored = [0, 1, 3, 0, 5, 4, 2, 0, 1];
let totalGoals = 0;
for (let i = 0; i < goalsScored.length; i++) {
totalGoals = totalGoals + goalsScored[i];
}
console.log("The team scored " + totalGoals + " goals this season.");
I, We, You Do
We can write the above loops inside our functions!Write a function totalGoals that will take in an array of goals (numbers) and return the total.
Entrance Test
So far, you learnt Javascript - how to store information in variables, how to use simple operators and adding comments to your code. Also, you have built an HTML/CSS website and link JS to the website. Now is time to test your knowledge with the following tests. Click to start!