Welcome to Prep JavaScript Course
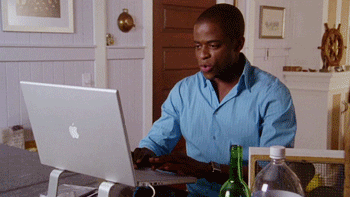
MODULE 11 - Intro to Javascript
Why Javascript
JavaScript is a high-level programming language that all modern web browsers support. It is also one of the core technologies of the web, along with HTML and CSS that you may have learned previously.
When it comes to programming, everyone has to start somewhere. In this program, you will focus first on learning JavaScript. Why? It's sometimes called the programming language of the web.
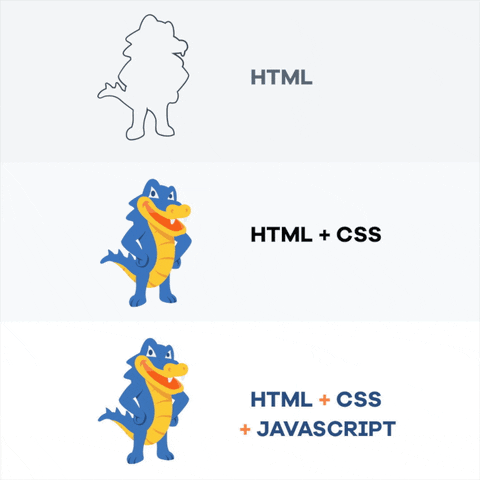
This section will cover basic JavaScript programming concepts, which range from variables, operators and comments.
Comments are lines of code that JavaScript will intentionally ignore. Comments are a great way to leave notes to yourself and to other people who will later need to figure out what that code does.
Adding comments
There are two ways to write comments in JavaScript:
Using // will tell JavaScript to ignore the remainder of the text on the current line:
// This is an in-line comment.
You can make a multi-line comment beginning with /* and ending with */:
This is how you use a multi-line comment /* */
I-We-You do exercise
Create a // style comment that contains at least five letters.
Create a /* */ style comment that contains at least five letters.
How to declare Variables
Variable names can be made up of numbers, letters, and $ or _, but may not contain spaces or start with a number.
The assignment operator, or “=” sign, is actually very misleading for anyone that is learning to code for the first time.
You are taught about the concept of the equals sign for your entire life in math class, but in JavaScript, the concept of a variable is actually is different.
By the end of this tutorial, you will understand the “=” in variable assignment, variables and more.
Reassigning Values to Variables
The let keyword allows you to create mutable variables whose values can be changed. If you used the const keyword, it would mean that the value is immutable and unchangeable. You'd learn more of const as you advance in JS.
In JavaScript, unlike math, you can simply assign a new value to the variable. Our days variable currently stands for the 7 days in a week. But what if we wanted it to stand for the 5 weekdays? Here is the code we could use.
IMPORTANT: In JavaScript we end statements with semicolons as seen above and below. Copy and paste in Repl.it
let days = 7; days = 5; console.log(days) // 5Using the let keyword above
In line 2, we create the days variable with a value of 7.
On line 4, we re-assign the value of the variable. It is now 5.
On line 6, the days truck arrives with the value of 5.

In the GIF above, line 4 puts a new value in the truck that is later used in line 6.
Here is what happens in line 6.

The variable days is not “equal” to anything! It merely carries around the value that you assign to it.
"Below is more efficient process than retyping that sentence multiple times throughout a program:" let quote = "If you believe, nothing is impossible"; console.log(quote); console.log(quote);I-We-You Do
Try it! Copy and paste the above in repl.it below:
NOTE: Variables allow you to store and track various items and values in your program.
No Take Home Assignment Here. Practice all above exercises
MODULE 12 - Converting data types
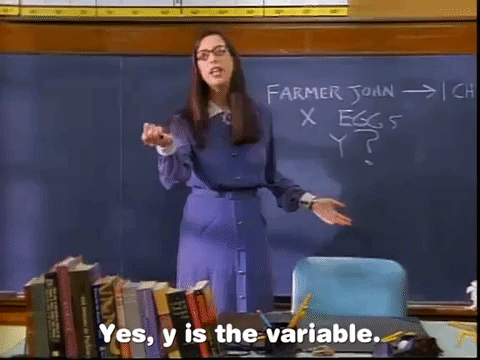
You can convert and combine data types. You can use the + operator in JavaScript to convert the numeric values into string values, which also combines the expressions into a string.
I-We-You Do
Copy and paste code below in repl.it.
let stringVar = 'I want to become a software engineer by '
let numVar = 2021
let combined = stringVar + numVar
typeof(combined) // => 'string'
console.log(combined) // => I want to become a software engineer by 2021
This Repl.it demonstrates that if you combine a number with a string using the + operator, the resulting value will be a string.
This process of automatic conversion is called coercion, which means the number was coerced into a string. But with all other operators, JavaScript does not convert numeric values into strings.
Linking HTML to Javascript
As you progress in your JS learning, you would need to connect your HTML code with JS code. In order for Javascript to work, you need to add < script src="index.js" > < /script> tags in your HTML codes
Overwrite Variables
To overwrite variables means that you can change the values of variables as needed. You can assign new values to your variables. See Example below and practice.
// Set the variable 'gaming' to "fun"
let gaming = "fun";
// Print the variable 'coding' to your console
console.log("gaming is " + gaming); //=> "gaming is fun"
I-We-You 2
Try it! Copy and paste the above in repl.it below:
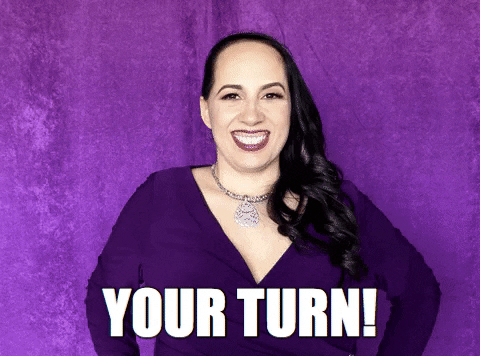
Now clear the code you pasted and declare a variable using let and output variables "gaming is addictive and obsessive" using console.log below:
// Set 'gaming' to "addictive and obsessive"
let gaming = "addictive and obsessive";
Take Home Practice
In the repl.it below:
Copy and paste the code given and print the variable 'coding' to your console
Use the variable below let coding = "cool";
// Use another variable below let coding = "trendy and fun";
//Expected Output for number 1 //-> "coding is cool"
//Expected Output for number 2 //-> "coding is trendy and fun"
Remember once you are done with your assignment, submit link of your repl.it code and your full name Submit Here
MODULE 13 - The different data types
A data type is a value that variables can have in a given programming language. Different kinds of data types can do different tasks.
Data types can be any of the following-
Number
String
Array
Object
Boolean
…boolean seems to be the easiest.
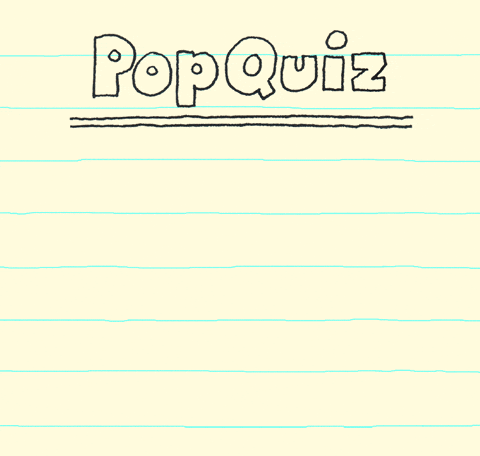
Example
let bool = true;
let bool= false;
The only two options for a boolean are true or false. And they are used in if() statements to decide which statement should be executed.
If Statement Example
if(true){
}else{
// if value is false, this block runs
}Within if statements, other variable values can evaluate to true or false. Once the value is used in the if() statement, JavaScript will evaluate whether it is true or false.
In JavaScript, the syntax for a string is a series of characters inside quotation marks. The quotes can be either single ' or double ".
But you must use the same type of quotation mark at the beginning and the end of a string—you can't start a string with a single quote and close it with double quotes.
I-We-You Do
Copy and paste code below in the repl.it
// declare a string with quotes
let city = "Seattle";
let state = "Washington";
How to camelcase
In JavaScript, when combining two words we use camelCase. A camelCased variable name starts with a lowercase letter for the first word, but every word after the first word begins with a capital letter. All the examples below are camelCased.
thisIsAnExample;
alsoThisIsAnotherExample;
andThisAlso;
Take Home Practice
1. Correct the following using camelcasing in a document:
let = "Salad";
let 2ndMeal = "Pasta";
let thirdMeal = "Jollof Rice;
let fourth = "Ice Cream";
2. In the repl.it below, Guess what would be printed
Remember once you are done with your assignment, submit link of your repl.it code and your full name Submit Here
MODULE 14 - Variable Assignment Operators
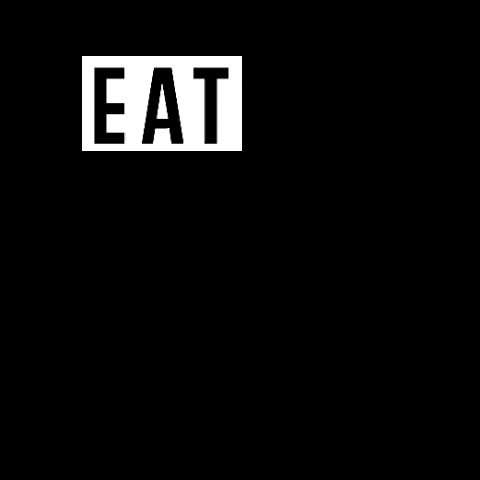
The name of the variable is on the left side of the assignment operator, while the value is on the right side.
In the code below is an example of an assignment operator.
We declared a variable (x). Value assigned to the variable (215). Console.log(x) prints the value in the variable x. (NOTE: console.log's are expressions for your code for now)
let x = 215;
console.log(x);// prints out 215
I-We-You Do
Addition, Mathematical and Assignment operators.
COPY AND PASTE THIS INTO THE script.js BELOW:
Addition Example
let y = 5 + 7; // y = 12
console.log(y);
Test these other Examples on repl.it.
//subtraction
let x = 8 - 6; x = 2 //change the numbers
console.log(x);
//multiplication
let j = 4 * 3; j = 12
console.log("j is " + j); // we can add words together, so change the letters!
//division
let b = 10 / 5; b = 2 console.log("y + b is " + (z + b));//change letters
I-We-You Do 2
// declare two variables here
// output your two variables using console.log()
Take Home Practice
1. In the repl.it below, copy and paste the code into your repl.it. How would you print the answer and what answers do you think it will print out?
let x = "42" + 7;
let y = "42" - 7;
2.What opening and closing tags do you use to link Javascript in your HTML code to make it work? Do your research, write your your answer in the email you send. Example: My answer is - < main> < /main> tags