MODULE 15 - Get to know strings & operators better
You'll likely recall that a string is made up of one or more characters—like letters, symbols, punctuation, and numbers—that are enclosed in single or double quotes. In JavaScript, a string is read as text.
let str = "nine"; // str is a string
let str = "9"; // str is a string
let str = 9; // str is a number (not good for a variable called 'str' to be a number!)
let str = nine; // error 'nine is not defined' --> the computer doesn't know how to deal with the word nine because it isn't in quotes
In your life as a developer, you'll work with strings all the time. Whenever a website deals with words or an app reveals text on a smartphone screen, strings are involved behind the scenes.
Here are some common scenarios that require advanced string features:
- Validating form submissions
- Injecting data into an HTML template
- Sorting a set of items alphabetically
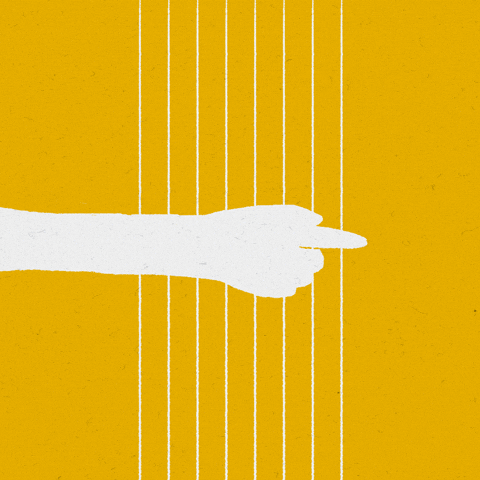
Strings show up all over the place! In fact, this text you're reading right now is probably represented in your computer as a string.
Previously we have used the code var myName = "your name";
"your name" is called a string literal. It is a string because it is a series of zero or more characters enclosed in single or double quotes.
Single quotes or double quotes?
Take a look at the following two lines of code.
let artist = 'Fionn Flowers Quartet';
let song = "Blues On The Corner";
Both artist and song are storing strings here. However, artist has single quotations, while song has double quotations. What's the difference?
The truth is, both options are valid in JavaScript—you can use either 'single quotes' or "double quotes" around a string. However, there are two important rules to keep in mind:
-
You can't mix quotations. In other words, you can't start with a single quote and end with a double, or vice versa. Following this rule, the line of code below is invalid.
let song = 'I Remember Clifford"
-
If you use double or single quotes within a string, you'll have to use the opposite to wrap the string. Consider the following example. Here, the string is wrapped in double quotes because there is a single quote inside the string, acting as an apostrophe.
let song = "I Got It Bad And That Ain't Good";
But let's make things a little simpler. For the time being, stick with double quotes, but use single quotes if you need to. Ultimately, it's more important to remain consistent than it is to choose a side.
String values in JavaScript may be written with single or double quotes, as long as you start and end with the same type of quote. Unlike some other programming languages, single and double quotes work the same in JavaScript.
doubleQuoteStr = "This is a string";
singleQuoteStr = 'This is also a string';
The reason why you might want to use one type of quote over the other is if you want to use both in a string. This might happen if you want to save a conversation in a string and have the conversation in quotes. Another use for it would be saving an tag with various attributes in quotes, all within a string.
conversation = 'Finn exclaims to Jake, "Algebraic!"';
However, this becomes a problem if you need to use the outermost quotes within it. Remember, a string has the same kind of quote at the beginning and end. But if you have that same quote somewhere in the middle, the string will stop early and throw an error.
goodStr = 'Jake asks Finn, "Hey, let\'s go on an adventure?"';
badStr = 'Finn responds, "Let's go!"'; // Throws an error
In the goodStr above, you can use both quotes safely by using the backslash \ as an escape character. Note
The backslash \
should not be confused with the forward slash /
. They do not do the same thing.
I, We, You Do
Change the provided string above to a string with single quotes at the beginning and end and no escape characters. Right now, the tag in the string uses double quotes everywhere. You will need to change the outer quotes to single quotes so you can remove the escape characters.
String Methods
Replace
Replace is a type of method and function that needs two pieces of information to work properly. Example below.
replace(brokenString, newString);
Note that replace() works to replace one string, or a part of a string, with another. In the code seen here, the first string in the parentheses, brokenString, is the string you will be replacing. The second, newString, is the string you want to put in. Play around with the REPL below to get a better sense of how this method works.
// First, let's see a normal string:
let description = "The hottest dance craze is the backslide.";
console.log(description);
// replace() replaces part of a string with something else
console.log(description.replace("backslide", "chicken"));
Leaving the original intact
Try this in the REPL above.
let word = "the word is not Capitalized CorrectlY.";
console.log(word);
word.toLowerCase();
console.log(word);
word.replace("the", "The");
console.log(word);
let lowerCased = word.toLowerCase();
let capitalized = lowerCased.replace("the", "The");
let corrected = capitalized.replace("not ", "");
// 'word' is still what it was at the start!
// the changed versions are stored in lowerCased, capitalized, and corrected
console.log(word);
Even with these methods applied, the original string " word " doesn't actually change. This might be surprising, so let's explore this further. Instead of changing the original string, the methods "turn into" a new value. These new values are being created and stored in the code, but they don't affect the original string. It's as if each piece of code is being swapped out for the result.
The example below, the comments next to each line explain what will be printed out to the console.
let loudText = "WHAT A GREAT DEVELOPER YOU CAN BE";
loudText.toLowerCase();
console.log(loudText); // WHAT A GREAT DEVELOPER YOU CAN BE
console.log(loudText.toLowerCase()); // what a great developer you can be
//Because there's no assignment operator = on the second line, the variable loudText is still holding onto "WHAT A GREAT DEVELOPER YOU CAN BE"
//which is entirely capitalized. To fix the code so that it logs the lowercase version
//the variable needs to be reassigned with a =, as seen below.
let loudText = "WHAT A GREAT DEVELOPER YOU CAN BE";
loudText = loudText.toLowerCase();
console.log(loudText); // what a great developer you can be
I, We, You Do
// Use the string methods you know to // 1. remove the extra whitespace at the beginning and end of the string, and // 2. capitalize all the letters // Make sure to assign your answer to the 'openAndPrep' variable let openAndPrep = " I like to use arrays! "; openAndPrep = openAndPrep.trim(); openAndPrep = openAndPrep.toUpperCase(); console.log(openAndPrep); // I LIKE TO USE ARRAYS!
Module 16 - Order of Operations
Order of operations
You might remember learning about PEMDAS in math class. PEMDAS is a simple tool that outlines the correct order of operations. It serves as a reminder of which mathematical procedures you should complete first, second, third, etc., when you're performing an equation. Fortunately, JavaScript follows the same order for these operators. But it also has other operators, like === and >.
- Operator precedence:
- Parentheses (())
- Exponents (**)
- Multiplication (*)
- Division (/)
- Addition (+)
- Subtraction (-)
Logical operators - The AND, OR, NOT
Often, you'll want your code to handle several conditions at once. You'll want it to consider a specific combination of conditions. For example, you might want to check that a user's password is long enough and that their chosen username is available.
That's where logical operators come in. They combine boolean values so that you can express more complex ideas in code, like this condition and that condition, this condition or that condition, and this condition but not that condition. The and, or, and not logical operators tell the code how to work with various pieces of information simultaneously.
In JavaScript, the three logical operators are written as follows: && (and), || (or), and ! (not). Take a look at these examples.
let youLoggedIn = true;
let youReturnedItem = false;
// test if both values are true
youLoggedIn && youReturnedItem; // => false
// test if either value is true
youLoggedIn || youReturnedItem; // => true
// invert the value of a variable
!youLoggedIn; // => false
!youReturnedItem; // => true
When you read the code above, you'll notice several things:
&& evaluates to true if the values on both sides are true.
|| evaluates to true if either one of the values is true.
! works on just one value, and gives the opposite of a value. Therefore, !true evaluates to false, and !false evaluates to true. And here is a summary in JavaScript.
// and (do both sides evaluate to true?)
true && true; // returns true
true && false; // returns false
false && true; // returns false
// or (does either side evaluate to true?)
true || true; // returns true
true || false; // returns true
false || false; // returns false
// not (return the inverse)
!true; // returns false
!false; // returns true
Relational operators
In addition to the operators discussed above, JavaScript has many other operators that allow you to test and assess the relationship between two items. Although you don't need to memorize all the relational operators, you should know that they exist as you build your JavaScript skills. That way, you can look them up when you need them! Remember, you can always refer to the documentation.
Check out this list of relational operators. What patterns do you see?
// Greater than - `>`
6 > 3; // true
1 > 11; // false
10 > 10; // false
// Less than - `<`
6 < 3; // false
1 < 9; // true
10 < 10; // false
// Greater than or equal to - `>=`
6 >= 3; // true
3 >= 11; // false
10 >= 10; // true
// Less than or equal to - `<=`
6 <= 3; // false
1 <= 9; // true
10 <= 10; // true
// Not equal - `!==` (opposite of ===)
6 !== 3; // true
1 !== 9; // true
10 !== 10; // false
Conditionals
If you have multiple conditions you want to check, you can use if ... else if ... else to check them one after the other.
Conditions and Decisions with Logical Operators
Logical operators can be used to form conditions in if..else statements.
let currentMoney; let laptopPrice; let laptopDiscountPrice = laptopPrice - (laptopPrice * .20) //Laptop price at 20 percent off if (currentMoney >= laptopPrice || currentMoney >= laptopDiscountPrice){ //Condition was true. Code in this block will run. console.log("Getting a new laptop!"); } else { //Condition was true. Code in this block will run. console.log("Can't afford a new laptop, yet!"); }I, You, We Do Exercise
Copy and paste above code. Play around with the REPL below to practice using logical operators.
let recentForecast = "rainy";
let recentWindSpeed = 30;
let holiday = true;
let currentDay = "Friday";
let message = "";
if (recentForecast === "rainy" || recentForecast === "snowing" && recentWindSpeed > 20) {
message = "Very Scary outside! Stay indoors!";
} else {
message = "It's not super scary and it might be nice";
}
console.log("Forecast for " + currentDay + " is: " + message);
if (recentForecast != "corona") {
console.log("There is too much corona today");
}
if (!holiday) {
console.log("You can't take today off!");
}
A Second Exercise
let loginCount = 3;
if (loginCount > 10) {
console.log("That's a lot of logins today, champ! Maybe give it a rest.");
} else if (loginCount > 0) {
console.log("Thanks for logging in!");
} else {
console.log("Don't forget to log in today");
}
Negation operator
You've seen so far how if you can use an if...else statement to create conditional logic. Anything that goes into an if needs to evaluate to true/false. By using the ! operator you can negate the expression. It would look like so:
if (!condition) {
// runs if condition is false
} else {
// runs if condition is true
}
Instead of only providing an else block, you can make another decision. In this example, there are three branches to the condition. See how you can use relational operators to perform this.
I, You, We Do Exercise
1. Copy and paste above code into the repl below:
2. Where do Equality Check (===), Not (!), And (&&), and Comparison (>) fit in this list? Try to add all four to the list. Use the code below to experiment and find out.
Hint: Try adding parentheses, changing the operators, and logging different values!
if (!5 - 4 > 2 === 2 * 10 / 5 === 4) {
console.log("the expression was truthy")
} else {
console.log("it was falsy");
}
console.log(!5)
console.log(!5 - 4)
console.log(4 > 2)
console.log(5 - 4 > 2)
console.log(4 > 2 === 2)
console.log(2 * 10 / 5)
console.log(2 * 10 / 5 === 4)
I, You, We Do Exercise
Copy and paste above code into the repl belowTernary expressions
if...else isn't the only way to express decision logic. You can also use something called a ternary operator. The syntax for it looks like this:
let variable = condition ? :
Below is a more tangible example:
let firstNumber = 20;
let secondNumber = 10
let biggestNumber = firstNumber > secondNumber ? firstNumber: secondNumber;
✅ Take a minute to read this code a few times. Do you understand how these operators are working?
The above states that
if firstNumber is larger than secondNumber
then assign firstNumber to biggestNumber
else assign secondNumber.
The ternary expression is just a compact way of writing the code below:
let biggestNumber;
if (firstNumber > secondNumber) {
biggestNumber = firstNumber;
} else {
biggestNumber = secondNumber;
}
Read more about operators on MDN and here
Module 17 - Intro to Arrays
Define Arrays
Arrays are collections of ordered items. In a sense, an array is like a storage box with many numbered items in it.
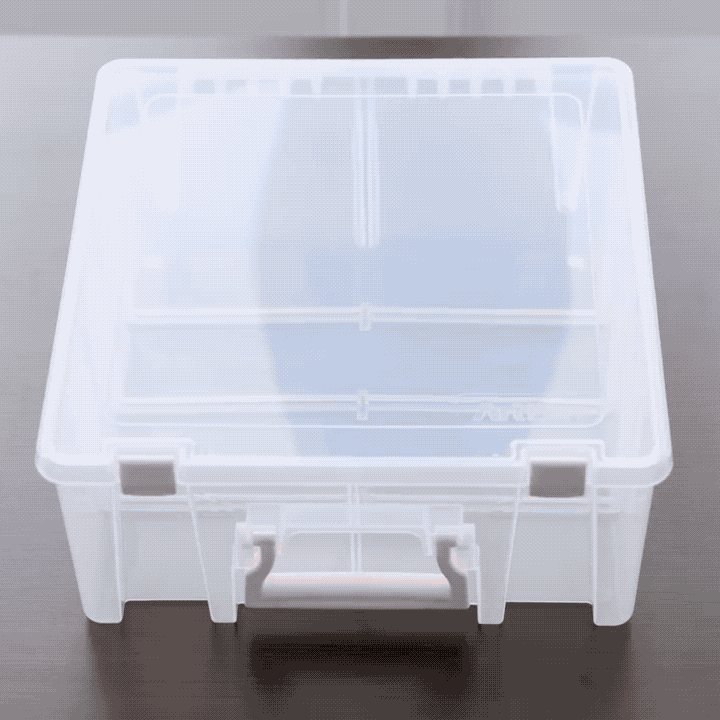
Imagine that you put something in compartment of this storage container. Whenever you come back to it, you'll find that everything is exactly as you had left it, with each part holding what you had originally put there. That's the idea behind an array—it helps you organize many items by storing every item in a specific place. In JavaScript, an array can have as many compartments or slots as you want!
Explain Array Syntax
With arrays, data is stored in a structure similar to a list. One major benefit of arrays is that you can store different types of data in one array.
✅ Arrays are all around us! The syntax for an array is a pair of square brackets.
let myArray = [];
This is an empty array, but arrays can be declared already populated with data. Multiple values in an array are separated by a comma.
let iceCreamFlavors = ["Chocolate", "Strawberry", "Vanilla", "Pistachio", "Rocky Road"];
The array values are assigned a unique value called the index, a whole number that is assigned based on its distance from the beginning of the array. In the example above, the string value "Chocolate" has an index of 0, and the index of "Rocky Road" is 4.
I, You, We Do Exercise
Copy and paste code into the repl below
let myFavoriteDishes = ["fries", "chicken", "shrimp"]; // an array of strings
let myBestNumbers = [3, 6, 13, 15, 21, 31]; // an array of numbers
let halfEmptyArray = myFavoriteDishes + myBestNumbers; // an empty array
let myFavoriteFruits = ["orange", "apple", "melon"]; // an array of strings
let myLuckyNumbers = [4, 8, 15, 16, 23, 42]; // an array of numbers
let emptyArray = myFavoriteFruits + myLuckyNumbers; // an empty array
console.log(myFavoriteFruits);
console.log(myBestNumbers);
console.log(halfEmptyArray);
As you may have noticed that a comma , separates each item in the array, but there's no comma after the last item. Take a look at these examples above.
Creating an array
You're ready to create an array in JavaScript. As always, play with the code in the Repl.it below; try making your own array and printing it out. And see what happens when you add two arrays together. Use the index with square brackets to retrieve, change, or insert array values.
let catAges = [3, 6, 9];
catAges[?] = ?; // a fourth cat, four years old
console.log(catAges);
2. Changing what's in an array
You've already learned that you can change what's in a variable by reassigning it. Refresh your memory by attempting what is below.
I, We, You Do
✅ Try it yourself! Use your browser's console to create and manipulate an array of your own creation. Copy and paste above code into the repl below
let iceCreamFlavors = ["Chocolate", "Strawberry", "Vanilla", "Pistachio", "Rocky Road"];
iceCreamFlavors[2]; //"Vanilla"
You can leverage the index to change a value, like this:
iceCreamFlavors[4] = "Butter Pecan"; //Changed "Rocky Road" to "Butter Pecan"
And you can insert a new value at a given index like this:
iceCreamFlavors[5] = "Cookie Dough"; //Added "Cookie Dough"
✅ A more common way to push values to an array is by using array operators such as array.push() - more on this later
Getting items from arrays
To get items from an array, you access an item in an array using bracket notation and the index of that item, which is that item's slot number. To do this, you use square brackets with the index inside, like [2]. This is easy to remember because you also create arrays with those square brackets [].
Here's an important detail that trips up many new developers. Arrays are zero indexed. That means that the numbering system starts at zero, not one. So if you want to retrieve the first item in an array, you must ask for the item in position 0. The first item is "zero items" from the beginning of an array, and is therefore in position 0.
Considering what you just learned about arrays, take a moment to return to your list of puppies in the example below. What do you notice?
let listOfPuppies = ["grey", "myka", "thunder"];
listOfPuppies[0]; // 'grey'
listOfPuppies[1]; // 'myka'
listOfPuppies[2]; // 'thunder'
Try out your new indexing skills in the REPL below. What happens if you try to access an index that doesn't have anything in it
I, You, We Do Exercise
1. Copy and paste below code into the repl below
let myFavoriteFruits = ["apple", "orange", "pineapple"];
// get items from myFavorite fruits
console.log(myFavoriteFruits[0]); //->
console.log(myFavoriteFruits[1]); // ->
console.log(myFavoriteFruits[2]); //->
// what will this do?!
//console.log(myFavoriteFruits[3]);
Commonly used array methods and properties
You've already explored some helpful methods for working with strings, like .replace() and .trim(). One of the benefits of arrays is that they come with their own methods to help you perform common tasks. In fact, there are more than thirty array methods! But for now, you can focus on the three most common ones:
.push():
This adds an item to the end of an array. It returns the new length of the array.
.pop():
This removes the last item from the end of an array. It returns the last item.
.length:
Just like the string tool .length, this gives the length of the array—in other words, the number of items. You'll notice that .length doesn't need parentheses. That's because it's technically a property and not a method.
Below learn more about .push(), .pop(), and .length.
I, You, We Do Exercise
Copy and paste above code into the repl below
let myFavoriteFruits = ["banana", "cherry", "mango"];
myFavoriteFruits.push("pear");
console.log(myFavoriteFruits);
myFavoriteFruits.push("banana");
console.log(myFavoriteFruits);
let theNewFruit = myFavoriteFruits.push();
console.log(theNewFruit);
console.log(myFavoriteFruits.length);
myFavoriteFruits.pop();
console.log(myFavoriteFruits);
console.log(myFavoriteFruits.length);
let theLastFruit = myFavoriteFruits.pop();
console.log(theLastFruit);
console.log(myFavoriteFruits.length);
2. To find out how many items are in an array, use the length property.
let iceCreamFlavors = ["Chocolate", "Strawberry", "Vanilla", "Pistachio", "Rocky Road"];
iceCreamFlavors.length; //5
Take Home Challenges
1. Complete the following on getting and reassigning array values
let listOfPuppies = ["shadow", "mocha", "ranger"];
let puppyAges = [0, 1, 2];
//A. access puppyAges at the right index to find shadow's age
let shadowAge = 0;
//B. mocha had a birthday! update her age to 2 in puppyAges
//C. There's a new puppy called 'holly' - add her to listOfPuppies
// don't forget to add holly's age (0) to the puppyAges array
2. Does the original array change?
let listOfPuppies = ["shadow", "mocha", "ranger"]; let puppyAges = [0, 1, 2]; //A. access puppyAges at the right index to find shadow's age let shadowAge = 0; //B. mocha had a birthday! update her age to 2 in puppyAges //C. There's a new puppy called 'holly' - add her to listOfPuppies // don't forget to add holly's age (0) to the puppyAges array
When you learned about the string methods .replace, .trim, .toUpperCase, and .toLowerCase, you discovered that the original string doesn't change when you use these methods. Is the same thing true for array methods? Copy and paste above code into the repl below
PLEASE ANSWER YES OR NO BELOW & EXPLAIN YOUR ANSWERS IN A DOCUMENT WHEN YOU SUBMIT
let myFavoriteFruits = ["banana", "cherry", "mango"];
console.log(myFavoriteFruits);
//A. does push change the array?
myFavoriteFruits.push("pear");
console.log(myFavoriteFruits);
//B. what about pop?
let last = myFavoriteFruits.pop();
console.log(myFavoriteFruits);
console.log(last);
Copy and paste your codes from the below exercises using comments and paste it in repl.it.Click links to get started
Use repl.it above to run these programs. Copy and paste your codes from the above exercises and paste it in repl.it. Submit Repl Links Here